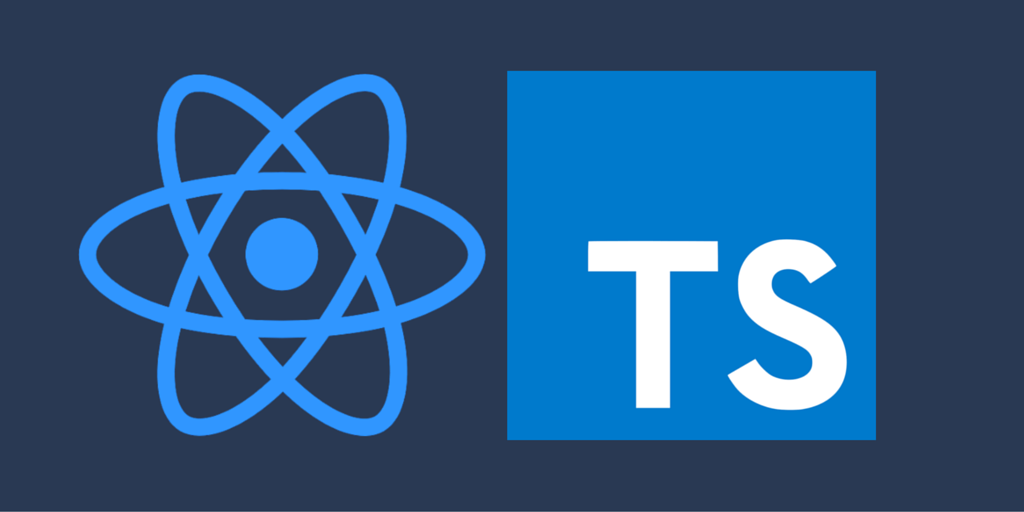
Using React with Typescript – crash course
since it’s relese in 2012, typescript has become more and more popular among developers and most companies and teams use typescript since it’s statically typed and teams can work together with less problems by catching errors and preventing bugs before building or deploying the project.
It is possible to use typescript with react thanks to react-scripts, and create react app also supports typescript and it enables you to setup typescript with react quickly.
In this article you will learn the basics of using ts with react.
Project Setup
setup your react typescript project with the following command:
npx create-react-app test-ts-app --template typescript
you will notice that the project files are different, create-react-app creates a tsconfig file for you which you can edit to config ts based on your need and the component files are tsx files which are ts files that support jsx.
so let’s see how can we take advantage of typescript in our react apps:
Functional Components and Props
while we are creating components we have to specify their types, the correct type for functional components is React.FC.
while without typescript we had to use PropTypes library to type component props, we can use ts interfaces to type props and pass the interface to the component type like this:
// Hello.tsx
export interface HelloProps {
name: string
}
const Hello: React.FC<HelloProps> = ({name}) => {
return (
<h1>Hello {name}</h1>
);
}
export default Hello;
since the name prop is required, if you forget to pass the prop, ts will show you an error in the ide:
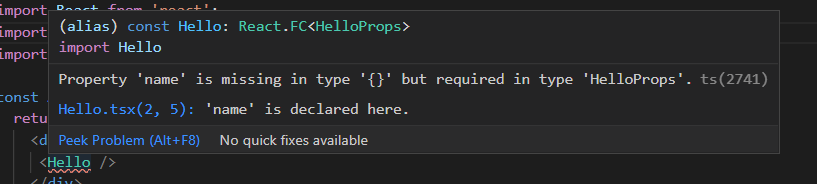
Ts will prevent any potential bugs if you forget required props or pass props with wrong types, without typescript you wouldn’t find out the problem before running the app.
States
Most times you don’t need to specify state types as ts finds out the state types implicitly, for example the count state here is implicitly types as number:
const [count, setCount] = useState(0);
But in some cases we have to explicitly define state types, for example the customer is null by default and it has a Customer type:
import React, {useState} from 'react';
interface CustomerType {
name: string,
age: number,
isMember: boolean
}
const User: React.FC = () => {
const [user, setUser] = useState<CustomerType | null>(null);
.
.
.
Events
when handling events, we have to specify event type to avoid errors:
const Name: React.FC = () => {
const [name, setName] = useState("");
const handleChange = (evt: React.ChangeEvent<HTMLInputElement>): void => {
setName(evt.target.value);
};
return (
<div>
<input type="text" onChange={handleChange} />
<p>name: {name}</p>
</div>
);
};
Conclusion
As we learned in this article, using typescript with react isn’t difficult at all it might feel boring to type everything at first but this more effort pays off in the long term.