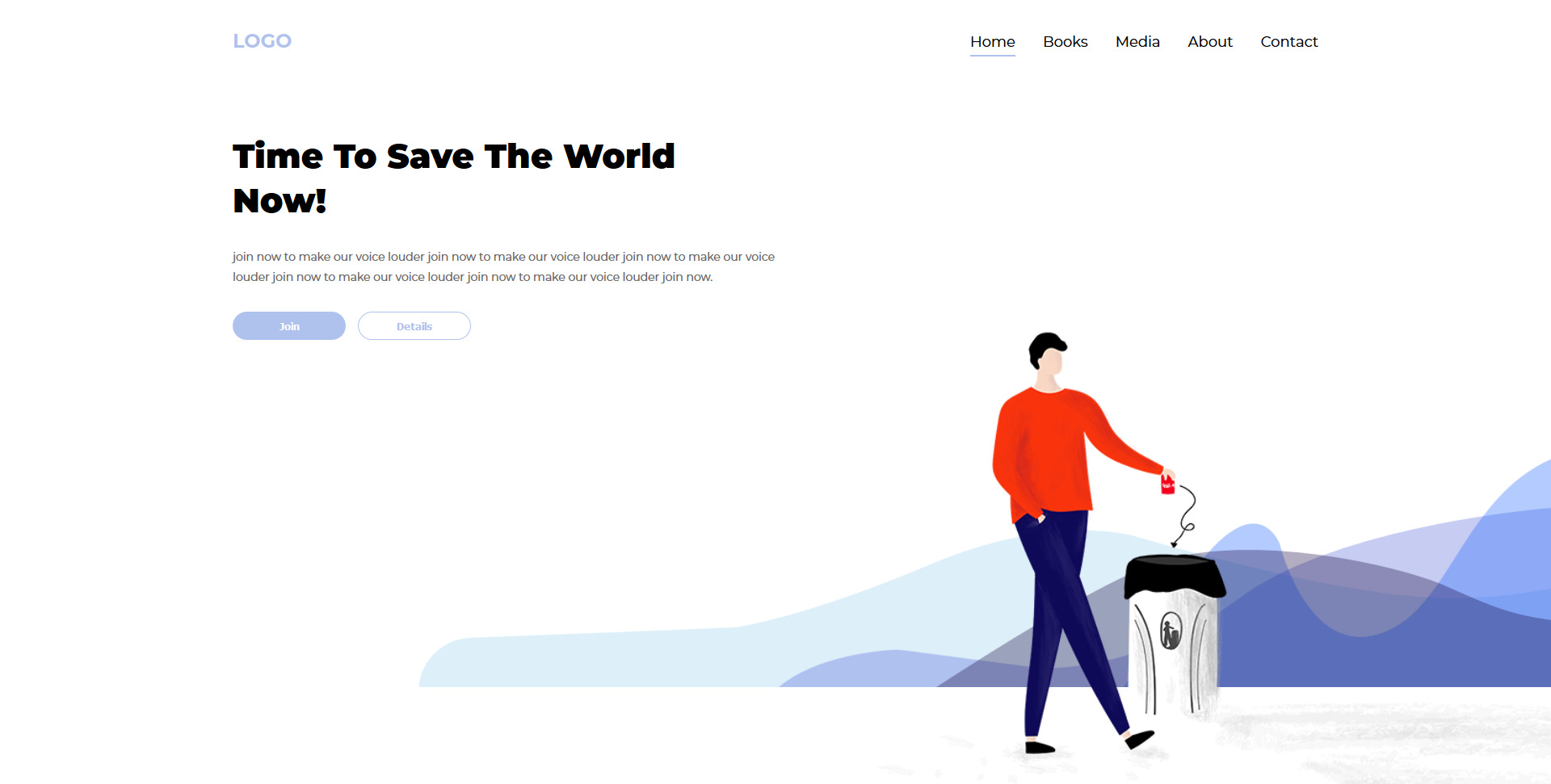
Mouse move parallax effect tutorial
adding more interactivity usually makes a website or application feel more professional and more interactive and you can improve user experience by doing that.
one of trending website interactive features is parallax effect with mouse move. so in today’s tutorial we’ll learn how to achieve that using html, css and javascript.
you can see the end result by clicking here: Demo
full code on codepen: codepen
the trick here is changing elements’ transform based on mouse position or device gyroscope sensor. while we could do that manually, for this tutorial I use an amazing pure javascript library, parallax.js
web page html structure:
after placing all other elements in the header, we’ll put image layers in the parallax container which is an absolute positioned div, data-depth attributes will set parallax layers:
.
.
.
<!----- parallax container and image elements, data-depth sets parallax layers-->
<div id="scene" data-relative-input="true">
<img src="img/bg1.svg" data-depth='0.9' width="100%" class="img-1"/>
<img src="img/bg2.svg" data-depth='0.7' width="100%" class="img-2"/>
<img src="img/bg3.svg" data-depth='0.5' width="60%" class="img-3" />
<img src="img/bg4.svg" data-depth='0.3' width="80%" class="img-4" />
<div class="ground" data-depth='0.2'></div>
<img src="img/man.png" data-depth='0.1' width="100%" class="img-5" />
</div>
<!----- scripts -->
<script src="parallax.min.js"></script>
<script src="script.js"></script>
</body>
</html>
initiate parallax effects:
in script.js we have to initiate parallax effects:
// initiate parallax effects
var scene = document.getElementById('scene');
var parallaxInstance = new Parallax(scene);
// you can change parallax options using parallaxInstance
position elements:
now we can see that the parallax effect is working but the img elements don’t have proper positions so we have to do that with css:
#scene{
position: absolute;
right: -10%;
bottom: 10%;
width: 80%;
}
.img-2{
margin-left: 18%;
}
.img-3{
margin-top: -3%;
margin-left: 50%;
}
.img-4{
margin-top: 7%;
margin-left: 30%;
}
.ground{
height: 300px;
width: 200%;
background: white;
margin-top: 19%;
margin-left: -100%
}
.img-5{
margin-top: -17%;
margin-left: 10%;
}
and now the elements are properly positioned and have parallax effect!