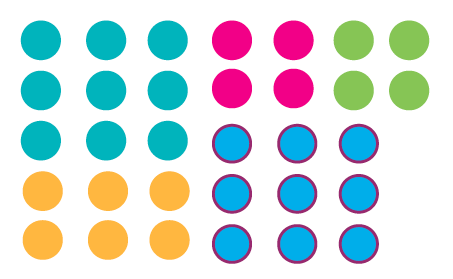
September 25, 2023
Efficiently Removing Duplicates from an Array of Objects in JavaScript
Dealing with arrays of objects is a common task in programming. Sometimes, you encounter situations where you need to remove duplicates from an array based on a specific key. In this article, we’ll dive into a JavaScript solution for this problem.
The Problem
Imagine you have an array of objects, and you want to eliminate duplicate entries based on a particular attribute. For example, you might have a list of products and want to remove duplicates based on their product IDs.
The Solution
Let’s create a JavaScript function called removeDuplicatesBasedOnKey
to tackle this problem efficiently. This function will take an array of objects and a key as parameters, ensuring that duplicates are removed while keeping the code simple and the time complexity low.
function removeDuplicatesBasedOnKey(array, key) {
const seen = new Map();
const result = [];
for (const item of array) {
const keyValue = item[key];
if (!seen.has(keyValue)) {
seen.set(keyValue, true);
result.push(item);
}
}
return result;
}
// Example: Removing duplicate products based on their 'id' key
const products = [
{ id: 1, name: "Product A" },
{ id: 2, name: "Product B" },
{ id: 1, name: "Product A" }, // Duplicate ID
{ id: 3, name: "Product C" },
{ id: 2, name: "Product B" }, // Duplicate ID
];
const uniqueProducts = removeDuplicatesBasedOnKey(products, 'id');
console.log(uniqueProducts); // Output: [{ id: 1, name: "Product A" }, { id: 2, name: "Product B" }, { id: 3, name: "Product C" }]
Conclusion
In this article, we’ve explored an efficient way to remove duplicate objects from an array in JavaScript, focusing on a specific key. The removeDuplicatesBasedOnKey
function can be a valuable addition to your coding toolkit, making tasks involving data manipulation more manageable.
By employing a Map
to keep track of seen key values, we maintain a low time complexity for the solution, ensuring it works well even with large datasets.