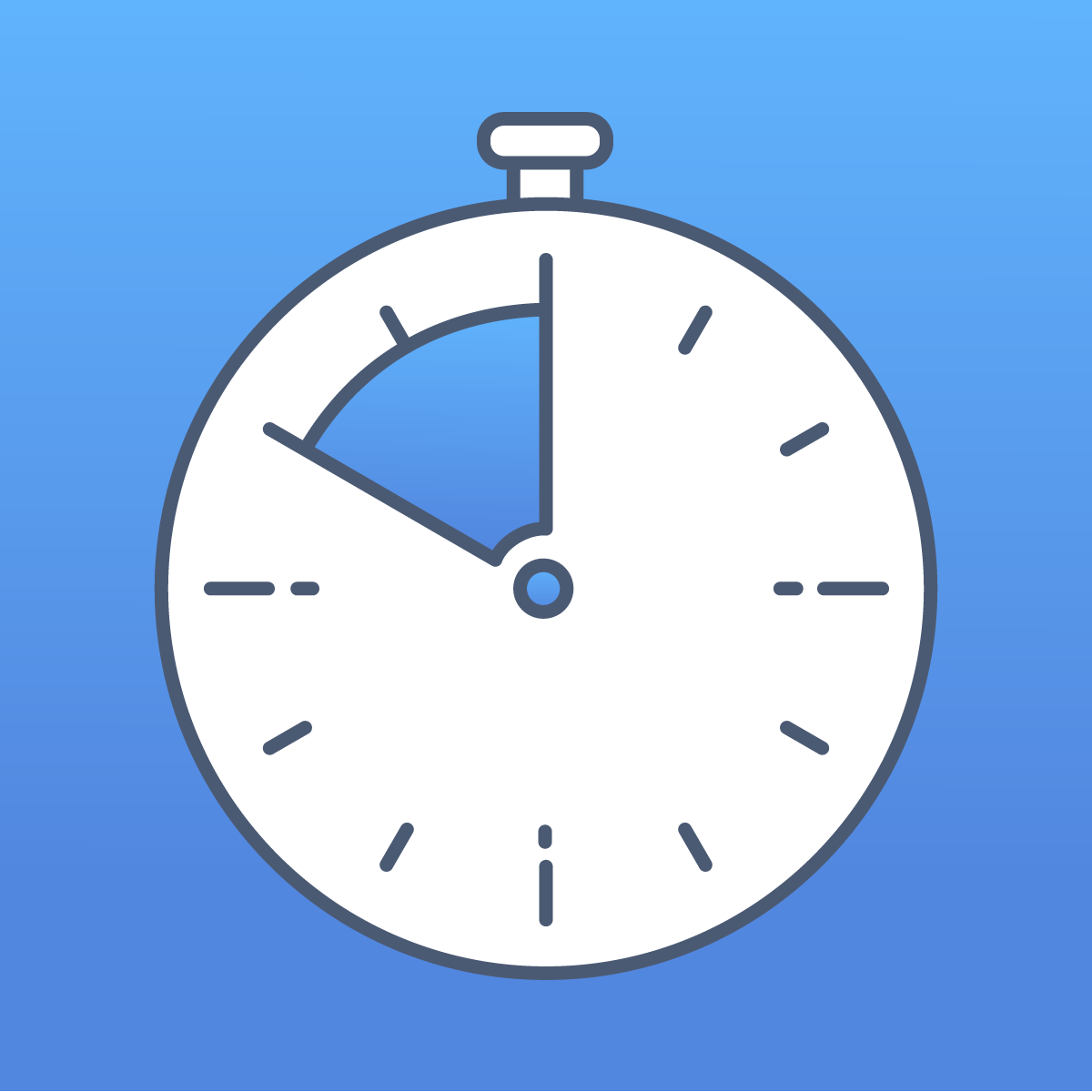
How to create a count down timer in react
One of the relatively common things that we might need in applications are timers like sale count down timers or exam or announcement count downs.
since such components are common and we may need to repeat the same tasks in different projects I created a custom react hook for handling count down timers as you know react hooks are the best way to share functionality in react you can learn how to create a custom hook in one of my previous posts
the hook that I created is “reactjs-countdown-hook” and it’s available on github
reactjs-countdown-hook is a simple count down timer hook for react that makes creating timers very easy this hook is very flexible and it accepts seconds and a function to run after the count down is over and it features formatted seconds, minutes, hours and days and it has functions for pausing, resuming and resetting.
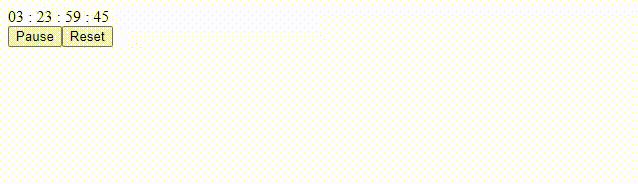
Installation
first of all install the package with the following npm command:
npm install --save reactjs-countdown-hook
Usage
the useTimer hook of “reactjs-countdown-hook” accepts initial remaining time as seconds and an optional callback function to run when the timer is over and the hook returns an object with the following properties:
- isActive: the state of the timer that shows if the count down is paused or it’s active – boolean
- counter: remaining time in seconds – number
- seconds: 00 formatted remaining seconds – string
- minutes: 00 formatted remaining minutes – string
- hours: 00 formatted remaining hours – string
- days: 00 formatted remaining days – string
- pause: a function to pause the count down – function
- resume: a function to resume the count down – function
- reset: a function to reset the count down to the given initial seconds – function
in the below example I have created a simple timer with 10 seconds initial remaining time and it alerts “times up!” when the count down is over:
import React from "react";
import { useTimer } from "reactjs-countdown-hook";
const App = () => {
const {
isActive,
counter,
seconds,
minutes,
hours,
days,
pause,
resume,
reset,
} = useTimer(10, handleTimerFinish);
function handleTimerFinish() {
alert("times up!");
}
return (
<div>
<div>{`${minutes} : ${seconds}`}</div>
<button onClick={() => (isActive ? pause() : resume())}>
{isActive ? "Pause" : "Resume"}
</button>
<button onClick={reset}>Reset</button>
</div>
);
};
export default App;
this hook let’s you easily create timers without extra states and functions and you can do it with any custom styles you want the timer is active by default since most timers are this way but you can use the pause and resume functions to determine when to start the timer by pausing it initially and resuming it later.