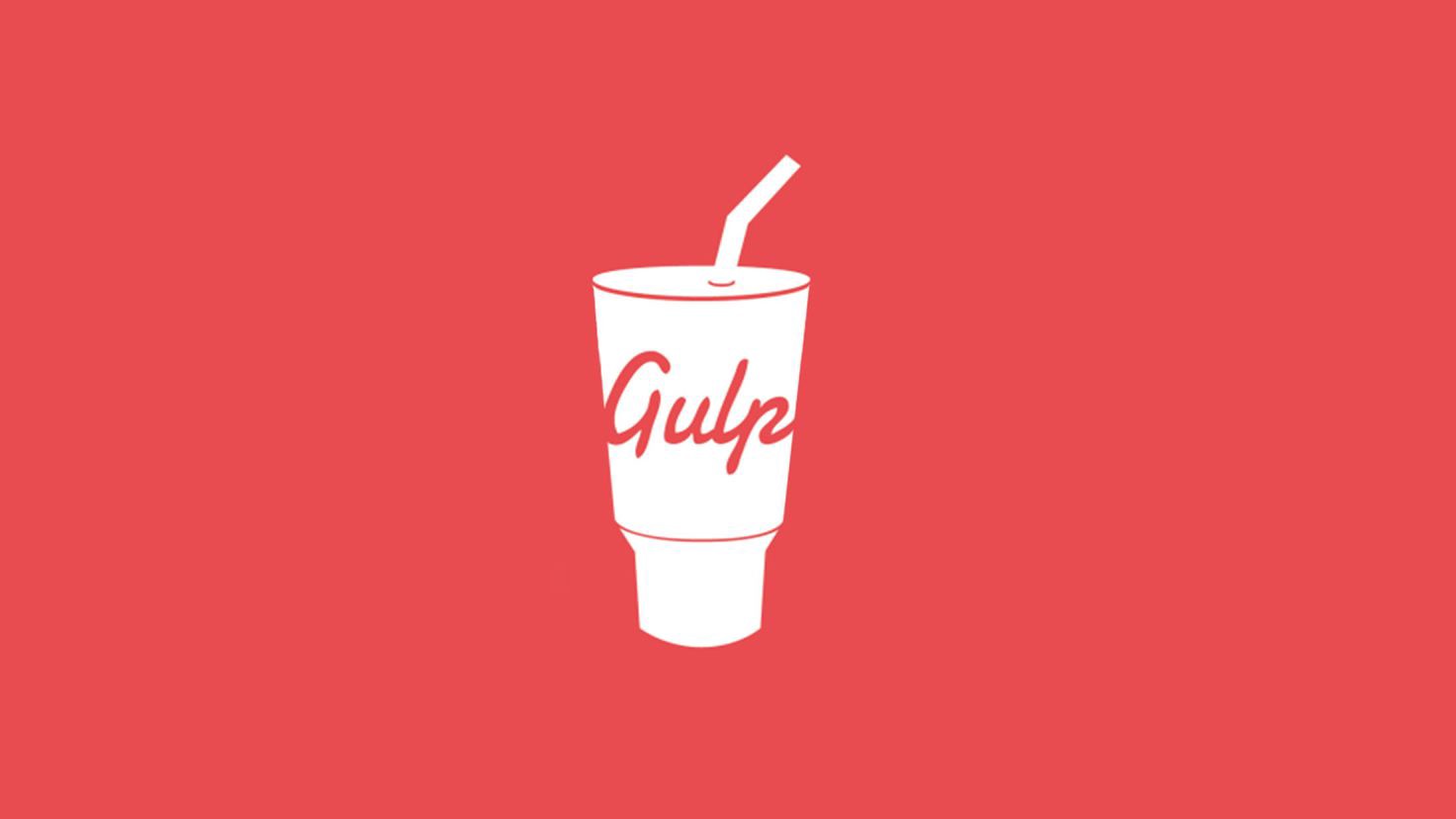
April 11, 2019
gulp beginner crash course
The front end development scene has changed a lot in recent years.
client side apps and websites just grow bigger and bigger and you may end up spending a lot of your precious time doing simple tasks like copying files and adding js and css files to your html files, can’t we make those tasks automatic?
using gulp and it’s many plugins we can make our lives easier, through this short tutorial we’ll see how to install and use gulp and do some common tasks step by step.
Installation
1-if you don’t have node js install it from here
2- create a new folder and open a cmd(terminal) window there and run this command:
npm init
after entering info, package.json file will be created, now install gulp:
npm i --save gulp
wait for npm to install the gulp package.
3- create a gulpfile.js file in the folder where package.json file is and open it.
Usage
1- import src, dest, series, watch from gulp:
const {src, dest, series, watch} = require('gulp');
2- create a src folder in the root folder where gulpfile is.
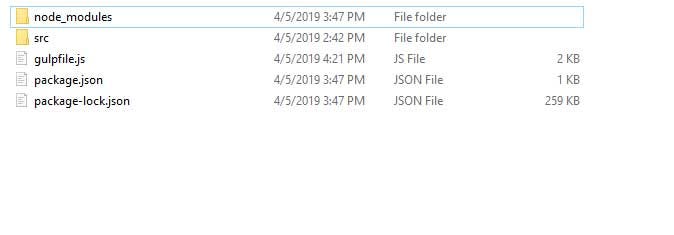
working with files
using src and dest functions we can copy files for example with this task we can copy all html files from src(development folder) to dist(final build folder):
plugins
gulp has a lot useful plugins, for example gulp-rename can rename files,
so first install it with this command: npm i gulp-rename and import it:
const rename = require('gulp-rename');
and change the previous function to rename html files:
function copyHTML(cb){
src('src/*.html')
.pipe(rename('index.html'))
.pipe(dest('dist/'))
cb();
}
using pipe we can chain multiple actions.
there are a lot of other plugins to use for other tasks like compiling sass and babel and their usage is just like the above function. just install, import and chain them in a correct order. for example the following task will babel, concat and minify all js files in one js file:
function compileJS(cb){
src('src/js/*.js')
.pipe(babel({
presets: ['@babel/preset-env']
}))
.pipe(concat('main.js'))
.pipe(uglify())
.pipe(dest('dist/'))
cb();
}
combining and Exporting tasks
calling each task one by one is tedious, using gulp’s series function we can run multiple tasks by running one task:
exports.build = series(copyHTML, compileJS);
by running gulp build all tasks will run.
watching files and running tasks automatically
but typing gulp build every time we want to see the changes isn’t an ideal way.
using gulp’s watch method, the specified tasks will run automatically:
function start(cb){
// we can specify multiple paths in an array:
watch(['src/main/*.html','src/pages/*.html'], copyHTML);
watch('src/js/*.js', compileJS);
cb()
}
exports.start = start;
so now the only command we have to run is:
gulp start
and whenever we change js and html files, the required task will run automatically.
and that’s all you need to know to start using gulp you can create as many as tasks and functions you like!