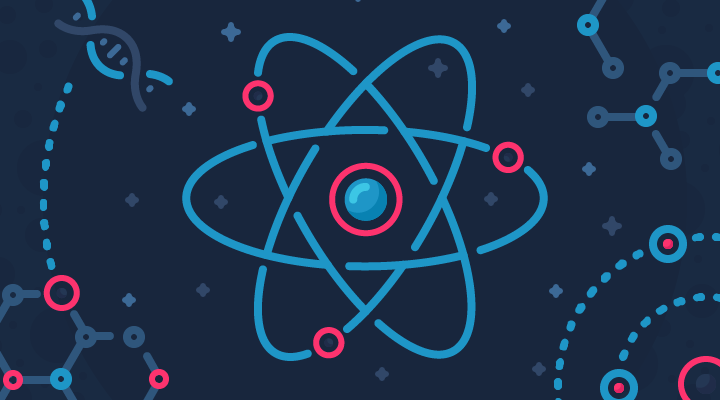
May 3, 2019
Preventing react components from updating
When I was working on a react project that used react context API I noticed that whenever I update the context, all components in the top most parent (which was App.js) updated while I just wanted one of them update, because the other components didn’t use data from context and had their own state.
So the solution to this problem which might happen when you use both a global state management (whether react context, redux or flux etc) and separate react states for each component, we have to:
1- Move all components that we don’t want to re render on global state change to a new parent component
so for example we have four components in App.js and we only want <Details /> component to re render on global state change, but we don’t want the other components re render. so instead of the code below we’ll move the other components to another component named Content.js:
// 1-
function App() {
const details = useContext(appContext);
return (
<>
<Header />
<Main />
<Footer />
<Details visible={details.visible} />
</>
}
// 2-
function App() {
const details = useContext(appContext);
return (
<>
<Content />
<Details visible={details.visible} />
</>
}
2- use useMemo hook in parent component’s return
the useMemo hook will tell react that this components shouldn’t update on global state change
function Content(){
return useMemo(()=>{
return(
<>
<Header />
<Main />
<Footer />
</>
)
},[])
the second argument of useMemo is a list of state variables that the component should update when they change, passing an empty array will prevent these components from re rendering.