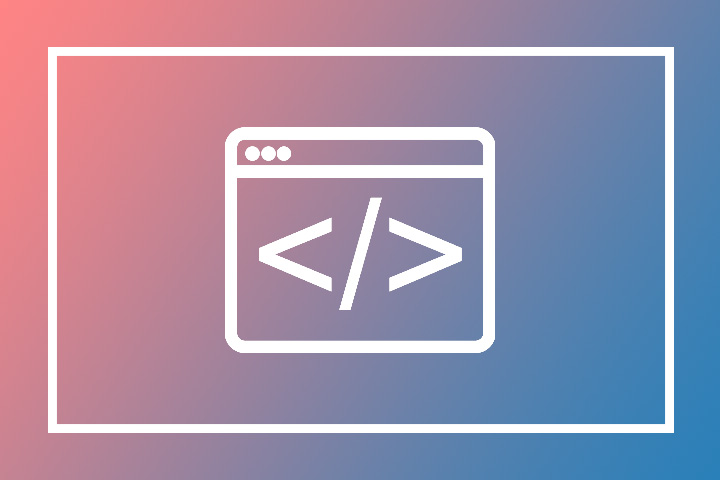
Lazy Loading Components in React
One of important performance optimization solutions is Lazy Loading. and it means instead of loading all resources on load we load resources on demand and only when they are needed or are visible. this technique usually reduces initial load time and render time significantly, specially when there are heavy components in the page.
When should you lazy load components?
If your react project is big or has many pages and components and the compiled main chunk javascript file is huge after building your application or there is a component in a page that is not visible by default (like a modal or collapse content) then it’s a good idea to lazy load some components.
note that you shouldn’t lazy load all components since it could lead to generating too many js chunk files and make your app less interactive and not smooth. so lazy load components only when it’s necessary or it improves the loading time and performance of your app.
How to lazy load components in react
In order to lazy load a component in react we have to:
1- use React’s lazy function and the import function instead of importing the component normally
2- wrap the lazy loaded component with the Suspense component
As mentioned above, one of the most common candidates for lazy loading are routes, in the below example the route components have been lazy loaded, by doing that a separate js chunk file would be generated for each route and it won’t be loaded until the user actually navigates to that route so the page loading time of the app will decrease (in some cases significantly) as the main chunk js file would be smaller:
import React, { lazy, Suspense } from "react";
import { Switch, Route, Link } from "react-router-dom";
const Home = lazy(() => import("./pages/Home"));
const About = lazy(() => import("./pages/About"));
const Articles = lazy(() => import("./pages/Articles"));
const App = () => {
return (
<div>
<ul>
<li>
<Link to="/">Home</Link>
</li>
<li>
<Link to="/about">About</Link>
</li>
<li>
<Link to="/articles">Articles</Link>
</li>
</ul>
<Suspense fallback={<div>Loading...</div>}>
<Switch>
<Route path="/" exact>
<Home />
</Route>
<Route path="/about">
<About />
</Route>
<Route path="/articles">
<Articles />
</Route>
</Switch>
</Suspense>
</div>
);
};
export default App;
Suspense has a fallback attribute and you can pass an element or a component to it, so while the js chunk file is being loaded the fallback will be displayed to the user. regularly a loading icon is used as the suspense fallback.