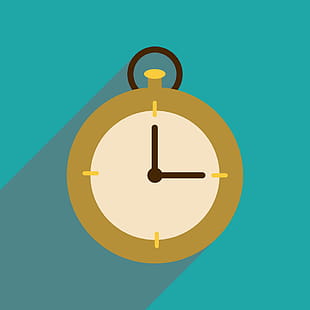
July 16, 2019
Calculating how much time does a js function take to run
In programming, performance is always one of the most important factors to consider, there are usually different solutions to a problem, but how to know which approach is the fastest? Is just getting the code to work enough?
In today’s short tutorial we’ll learn how to calculate how much time a function takes to run so that we can prevent potential lags and freezes.
JS has a reliable built in function for doing that and that is performance.now()
imagine we need a function to calculate sum of all numbers from 1 to n
the first solution that comes to mind is doing that with a for loop, let’s calculate how much does this function take to run:
function sumNumber(n) {
let sum = 0;
for (let i = 1; i <= n; i++) {
total += i;
}
return sum;
}
var t1 = performance.now();
sumNumber(1000000000);
var t2 = performance.now();
console.log(`Time Elapsed: ${(t2 - t1) / 1000} seconds.`) //almost 0.9s
so by subtraction t1 from t2 we find out that this function takes about 0.9 seconds to run which isn’t good.
now let’s try a different solution and use a mathematical formula instead of looping for 1 billion times!
function sumNumberNew(n) {
return n * (n+1) / 2;
}
var t1 = performance.now();
var gg = sumNumberNew(1000000000);
var t2 = performance.now();
console.log(`Time Elapsed: ${(t2 - t1) / 1000} seconds.`) //almost 0.00001s!
so whenever we doubt that if a function we wrote runs fast enough we can do a quick check by running performance.now before and after running the function.